👍 go run linked_list.go
5 3 1
last node: 1
5 3 1 10
node with 3 found: true
node with 4 found: false
5 3 1 6 10
package main
import("fmt")
type Node struct {
property int
nextNode *Node
}
type LinkedList struct {
headNode *Node
}
func(l *LinkedList)AddToHead(p int){
var n = Node{}
n.property = p
n.nextNode = l.headNode
l.headNode = &n
}
func(l *LinkedList)IterateList(){
var n *Node
for n = l.headNode; n != nil;
n = n.nextNode {
fmt.Print(n.property, " ")
}
fmt.Println()
}
func(l *LinkedList)LastNode() *Node{
var n, lst *Node
for n = l.headNode; n != nil;
n = n.nextNode {
if n.nextNode == nil {
lst = n
}
}
return lst
}
func(l *LinkedList)AddToEnd(p int){
var n = &Node{}
n.property = p
n.nextNode = nil
var lst *Node
lst = l.LastNode()
if lst == nil {
l.headNode = n
} else {
lst.nextNode = n
}
}
func(l *LinkedList)NodeWithValue(
p int) *Node{
var n, nWth *Node
for n = l.headNode; n != nil;
n = n.nextNode {
if n.property == p {
nWth = n
break
}
}
return nWth
}
func(l *LinkedList)AddAfter(
nP int, p int){
var n = &Node{}
n.property = p
n.nextNode = nil
var nWth *Node
nWth = l.NodeWithValue(nP)
if nWth != nil {
n.nextNode = nWth.nextNode
nWth.nextNode = n
}
}
func main() {
var l LinkedList
l = LinkedList{}
l.AddToHead(1)
l.AddToHead(3)
l.AddToHead(5)
l.IterateList();
fmt.Println("last node:",
l.LastNode().property)
l.AddToEnd(10)
l.IterateList();
fmt.Println("node with 3 found:",
l.NodeWithValue(3) != nil)
fmt.Println("node with 4 found:",
l.NodeWithValue(4) != nil)
l.AddAfter(1, 6)
l.IterateList();
}
ch3 Linear Data Structures p99/83 of
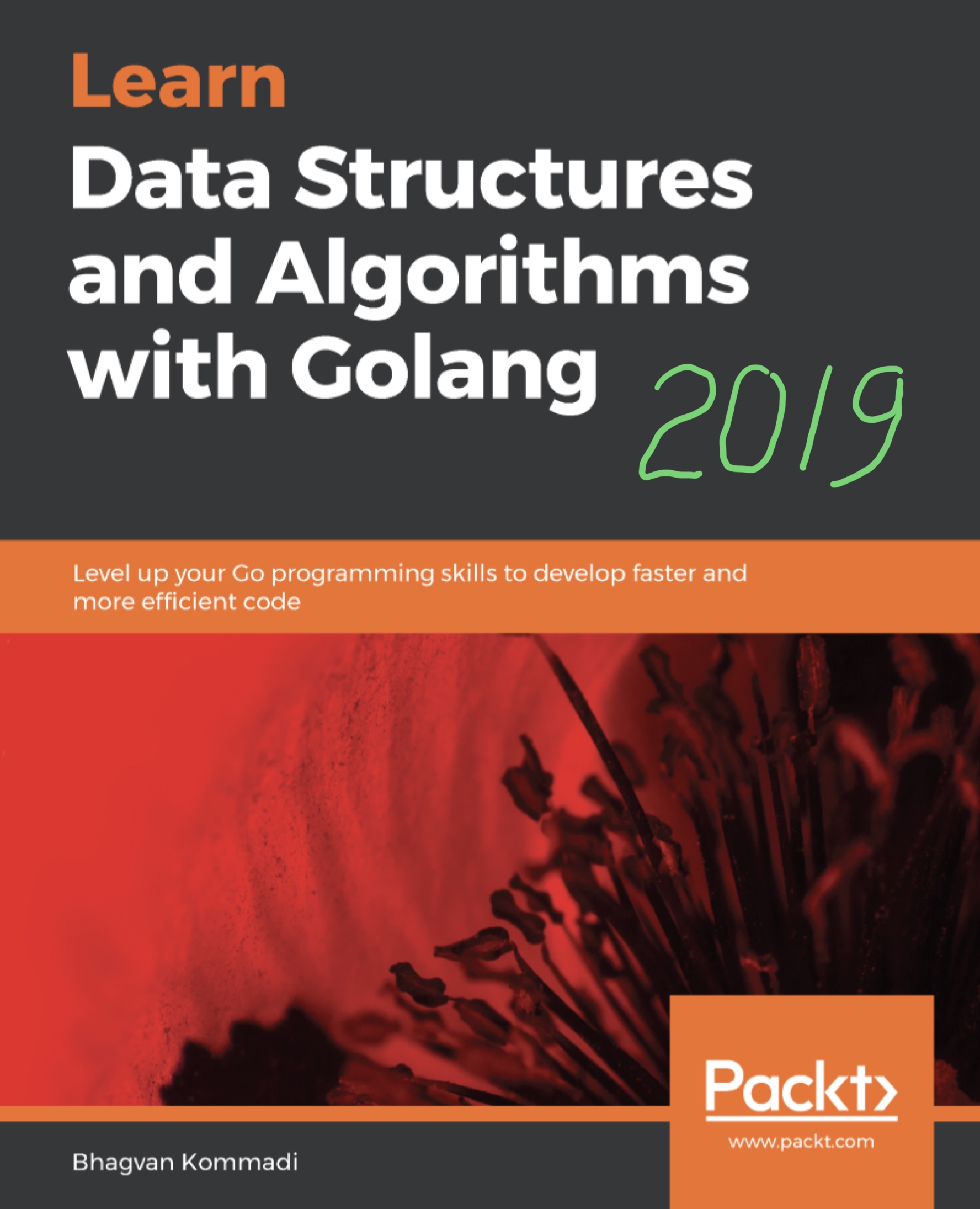